Developer Support
Set Up an Embedded Script Component
6 min read
·
Last updated on May 30, 2023
Setting up an embedded script app in the Wix Developers Center enables you to insert custom script tags to the <head> tag of your users' websites.
Here's what you'll need to do:
Add an embedded script component
- Create a new app, or log in to an existing app in the Wix Developers Center.
- Go to Components in the side menu under Build Your App.
- Click Add Component > Website Component.
- Select Embedded Script and click Add Component.
- Name your component – it can only contain letters and the hyphen (-) character.
- Select a Script Type – this is used by the Cookie Consent Banner tool to determine whether site visitors consent to having your script run during their visit.
Site owners can choose to give site visitors the option to opt out of cookies and third-party scripts, by type. The four available types are:
Essential: Enables site visitors to move around the website and use essential features like secure and private areas crucial to the functioning of the site.
Functional: Remembers choices site visitors make to improve their experience (e.g. language).
Analytics: Lets site owners understand how site visitors use their website (e.g. which pages they visit), to provide statistics on how their website is used, improve the website by identifying any errors, and performance issues.
Advertising: Lets site owners collect information to help market their products, such as data on the impact of marketing campaigns, re-targeted advertising, etc.
About types
An embedded script component can't be saved without a type. If your script falls into more than one type, choose the option closest to the bottom of the list above. For example, if your script has Advertising and Analytics aspects, choose Advertising as its type. It's unlikely that you'll need to mark it as Essential – if you think you need to use this, please get in touch with us.
7. Add the script to inject, with any relevant dynamic key parameters.
8. Set up a dashboard component in the Wix Developers Center. This lets users set up the embedded script and customize it later on.
9. Generate an Access Token. You'll need OAuth access set up for this to work.
10. To finish you'll need to embed your script and update the values of dynamic parameters in each app instance with the Embedded Scripts API.
1
POST https://www.wixapis.com/apps/v1/scripts
Request body:
1 2 3 4 5 6 7
{ "properties": { "parameters": { "KeyName123": "Value123" } } }
Don't forget to include an 'Authorization' header with the Access Token generated on step #9.
Test your app
It's important to test your app on your site to make sure that everything's connected and working properly. If it is, you should see your script injected to the site with the appropriate parameter in place.
Note:
Embedded script apps can only be added by the owners of Premium Wix sites with connected domains and a valid SSL certificate. If you want your embedded script app to be available to the owners of free Wix sites, you need to make a request.
Dynamic key parameters
You may choose to embed dynamic parameters in your script, if you have any custom keys per user. For example:
1
<script id="my-script" src="https://cdn.example.com/pixel.js?id={{KeyName123}}" async='true'></script>
Dynamic parameters must be:
- Wrapped in double curly braces.
- Within a quote (") in order to avoid code evaluation.
- Set using the Embedded Scripts API.
Important:
If you add, remove, or change a dynamic key, users will need to update your app to receive the latest version of the embedded script. This means that any users that don't update your app will remain on older versions of the script.
Note:
You can also inject script into placeholders provided by Wix Stores.
Important:
Test your app on your site to make sure everything is connected and working properly. If everything it working properly, you should see your script injected to the site with the appropriate parameter in place.
The Embedded Script SDK
Note:
This SDK isn't related to or dependent on the old JS SDK.
The embedded script SDK allows apps with embedded script components to:
- Listen to predefined Wix events
- Report and listen to custom events
Listening to a predefined Wix event
Add this code to your embedded script component, and make sure to point to the events you're interested in. You'll also need to change 'app-ID' to your own unique App ID for the code to work.
1 2 3 4 5 6 7 8 9 10 11 12
<script id='my_app_js' src='https://cdn.domain.com/pixel.js?id={{pixel_id}}' async='true'></script> <script> window.wixDevelopersAnalytics.register('app-ID', function report(eventName, data) { switch(eventName) { case 'pageview': callMyPageViewFunction(); break; case 'addToCart': callMyAddToCartFunction(); break; }); </script>
Predefined Wix Events
- addProductImpression: When a user views a product.
- clickProduct: When a user clicks on a product.
- viewContent: When a key page is viewed.
- addToCart: When a user adds a product to the shopping cart.
- removeFromCart: When a user removes a product from the shopping cart.
- initiateCheckout: When a user starts the checkout process.
- startPayment: When a user starts the payment process.
- addPaymentInfo: When a user saves payment information.
- checkoutStep: When a user completes a custom checkout step.
- purchase: When the checkout process is complete.
- lead: When a user subscribes to a newsletter or submits a contact form.
Reporting a custom event
Call a trigger event, passing the following properties:
- eventName
- eventData / eventParams – an object with the data to be passed
1
window.wixDevelopersAnalytics.triggerEvent(<'FORMSUBMIT'>, {name: <'TEST'>})
Reporting and listening to a custom event
Add this code to your embedded script component, and make sure you point to your custom events. You'll also need to change 'app-ID' to your own unique App ID for the code to work.
1 2 3 4 5 6 7 8 9 10
<script> window.wixDevelopersAnalytics.register('app-ID', function report(eventName, data) { console.log('eventName: '+eventName); }); setTimeout(function(){ window.wixDevelopersAnalytics.triggerEvent('newEvent', {eventName: 'eventName'}) }, 5000); </script>
On-ready event
To make sure you aren't calling the SDK before it is fully initiated, here's an on-ready event you can listen to. You'll also need to change 'app-ID' to your own unique App ID for the code to work.
1 2 3 4 5 6 7
window.addEventListener('wixDevelopersAnalyticsReady', registerListener) function registerListener(){ window.wixDevelopersAnalytics.register('app-ID', function report(eventName, data) { console.log("New Event: "+eventName+" | Data: "+JSON.stringify(data)) }); };
Response data
The returned data will vary based on the specific event, but will always include the following:
- isPremium: true if the site owner has a premium plan, false if they have a free plan.
- uuid: The site owner's unique ID in Wix.
- msid: The unique ID of the Wix Site.
Embedded Script Placeholders
Wix Stores provides two placeholders where you can inject your embedded scripts:
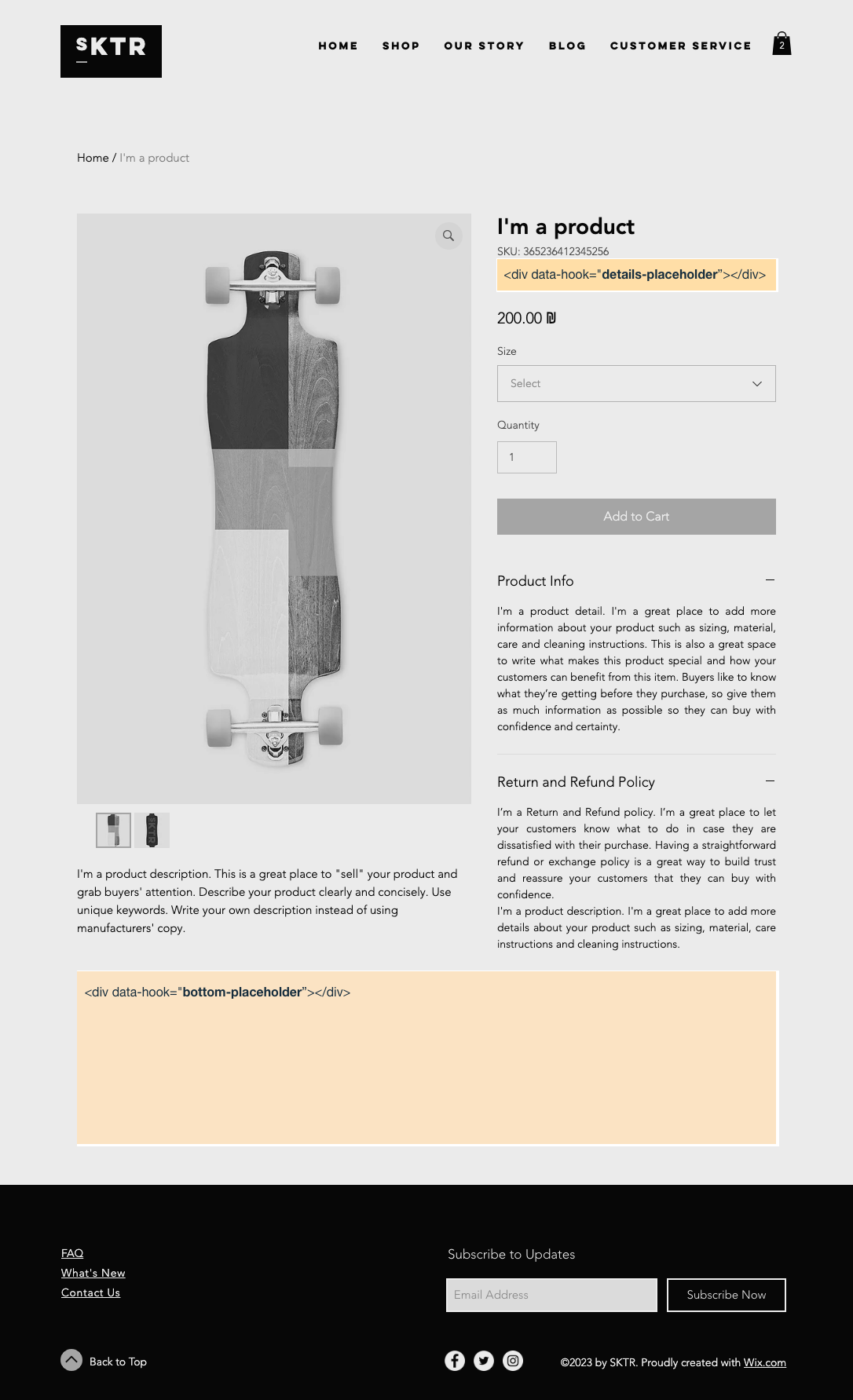
Below the product SKU, you should find:
1
<div data-hook="details-placeholder"></div>
And, below the product page, you should find:
1
<div data-hook="bottom-placeholder></div>
The placeholders are empty divs with data-hooks that allow you to add content to these specific page locations.
To inject data into one of these placeholders, append your DOM element to the relevant placeholder once the page is loaded.
To inject data into one of these placeholders, append your DOM element to the relevant placeholder once the page is loaded.
Important:
Make sure you are appending your DOM elements – don't replace the content, in case other apps are using the same placeholder on a site.
Was this article helpful?